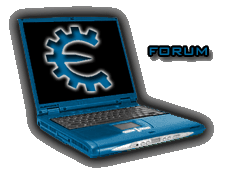 |
Cheat Engine The Official Site of Cheat Engine
|
View previous topic :: View next topic |
Author |
Message |
bknight2602 Grandmaster Cheater
Reputation: 0
Joined: 08 Oct 2012 Posts: 582
|
Posted: Fri Feb 28, 2025 11:09 am Post subject: Next after error |
|
|
Are there commands to either continue next or jump next in LUA after an error is encountered, much like ON ERROR RESUME NEXT IN VBA?
|
|
Back to top |
|
 |
AylinCE Grandmaster Cheater Supreme
Reputation: 35
Joined: 16 Feb 2017 Posts: 1483
|
Posted: Fri Feb 28, 2025 3:48 pm Post subject: |
|
|
Of course it is possible. Because you still need to predict the locales that may give an error.
So you can leave a "check" on the entity there, see the result, and "cancel" or "continue" in case of an error.
Code: | local myCheck = "abcde"
if myCheck:find("f") then
print("success!")
else
print("not found!")
end
local myCheck1 = "abcdef".."ghi"
if myCheck1:find("i") then
print("success!")
else
print("not found!")
end |
-- or "pcall" options:
Code: | local status, err = pcall( function ()
-- your lua codes ...
end) print(err, status) |
--use:
Code: | local status, err = pcall( function ()
local myCheck = "abcde"
if myCheck:find("f") then
print("success!")
else
print("not found!")
end
--local myCheck1 = "abcdef".."ghi" -- error line ...
if myCheck1:find("i") then
print("success!")
else
print("not found!")
end
end) print("my pcall:", err, status) |
Note:
"Skipping the wrong line"!
This could be an escape situation that will prevent learning, development, and advanced application..
Probably including @DB (Of course, this is my opinion.) no creator would want a student or implementer to make such a request to be a "code escape".
Finding, fixing or developing the error will allow you to get better results.
_________________
|
|
Back to top |
|
 |
bknight2602 Grandmaster Cheater
Reputation: 0
Joined: 08 Oct 2012 Posts: 582
|
Posted: Sat Mar 01, 2025 12:14 pm Post subject: |
|
|
The errors are in a do loop that has a variable set of conditions that are changed by initial settings. The last seven are the culprits, they change with the variability of the start. I "fixed" it by excluding the last seven from a do loop.
|
|
Back to top |
|
 |
AylinCE Grandmaster Cheater Supreme
Reputation: 35
Joined: 16 Feb 2017 Posts: 1483
|
Posted: Sat Mar 01, 2025 2:41 pm Post subject: |
|
|
This is much better.
Adding checks that test the existence or content of the locale beforehand keeps the code more stable in future uses.
The questions we ask and the answers we give are in the nature of "Archive".
Thanks for your feedback on how you fixed it.
_________________
|
|
Back to top |
|
 |
bknight2602 Grandmaster Cheater
Reputation: 0
Joined: 08 Oct 2012 Posts: 582
|
Posted: Wed Mar 12, 2025 11:47 am Post subject: |
|
|
OK, Now I have another question. I have a function that generates a string variable. Now I need to pass this string variable to another function but the variable needs to be passed as variable say x, for use in this second function.
something like this:
function one creates string variable "test" Now "test" needs to be sent to a different function that uses any variable string sent to it as x.
function (2 x, do something)
How is this worded?
|
|
Back to top |
|
 |
AylinCE Grandmaster Cheater Supreme
Reputation: 35
Joined: 16 Feb 2017 Posts: 1483
|
Posted: Wed Mar 12, 2025 3:19 pm Post subject: |
|
|
If we proceed through code examples, we will find more options, for questions and answers.
Examine the code below, let's discuss what and how it should be by giving examples.
exp: 1
Code: | local x = "1"
function fnc2(x1, opt)
local x2 = "your x: " .. x
return x2, x1, opt
end
function fnc1(x)
opt = " true"
x = x.."2"
local x1 = {fnc2(x, opt)}
print(x1[1], x1[2], x1[3])
end
x3 = " My test x; "
fnc1(x3) |
exp: 2
Code: | -- The first function creates a string variable
function functionOne()
local test = "This is a test string"
return test
end
-- The second function takes a string variable as input and processes it as 'x'
function functionTwo(x)
print("The value of x is: " .. x)
end
-- Call the first function, retrieve its output, and pass it to the second function
local result = functionOne()
functionTwo(result) |
_________________
|
|
Back to top |
|
 |
bknight2602 Grandmaster Cheater
Reputation: 0
Joined: 08 Oct 2012 Posts: 582
|
Posted: Fri Mar 14, 2025 1:53 pm Post subject: |
|
|
OK then this might work
Code: | Trigger(OBJECT_CAPTURE_TRIGGER, "empathabbey", "empathabbeycaptured");--Name of object or could be an assigned variable, but in this case it is the name Second part is a function if the capture is made.
end;
|
Furher forward in the code
Code: |
function empathabbeycaptured(oldOwner,newOwner,heroName)
t_empathabbey = newOwner;Owners-- are #' not needed for my question
BuildTown(empathabbey)-- here is the question in this function normally has the object name in parentheses but I jest sent the name without the parentheses. how would I
so how would
end;
|
How do I transform any name without parentheses to one with the name in parentheses?
|
|
Back to top |
|
 |
AylinCE Grandmaster Cheater Supreme
Reputation: 35
Joined: 16 Feb 2017 Posts: 1483
|
Posted: Fri Mar 14, 2025 6:21 pm Post subject: |
|
|
For a function to work correctly in Lua, the parameter passed to the function must be either an object, a string, or a variable. If empathabbey is not defined, BuildTown(empathabbey) returns an error.
If an Object is Used:
Code: | function empathabbeycaptured(oldOwner, newOwner, heroName)
-- Assigning empathabbey to an object or string
local empathabbey = {name = "Empath Abbey", owner = newOwner}
-- We send the object to the function
BuildTown(empathabbey)
end
|
If empathabbey actually represents just a string:
Code: | function empathabbeycaptured(oldOwner, newOwner, heroName)
-- empathabbey is assigned as a string
local empathabbey = "Empath Abbey"
-- We send the string to the function
BuildTown(empathabbey)
end
|
If in Lua the function needs to be called without parentheses (for example, if a string literal is passed), it can be written as follows:
Code: | BuildTown "Empath Abbey"
|
If empathabbey is defined as an object, variable, or string and needs to be passed outside the parentheses,
give it a variable outside the parentheses:
Code: | local empathabbey1 = "This strings.."
local nextIndex = 1
function BuildTown(empathabbey)
if empathabbey~="" then -- Test for alternative parameter (Local)..
if nextIndex==1 then
print("nextIndex " .. nextIndex .. " : " .. empathabbey)
elseif nextIndex==2 then
print("nextIndex " .. nextIndex .. " : " .. empathabbey)
end
else -- use: empathabbey1
print("nextIndex " .. nextIndex .. " : " .. empathabbey1)
end
nextIndex = tonumber(nextIndex) + 1
end
empathabbey = "Whatever happens.."
BuildTown(empathabbey) -- nextIndex 1 : Whatever happens..
empathabbey = empathabbey1
BuildTown(empathabbey) -- nextIndex 2 : This strings..
empathabbey1 = "Something new.."
BuildTown("") -- nextIndex 3 : Something new.. |
Of course, if this is a function that is processed and runs in the game code, not in your script code, there may be different interpretations.
However, if this is a code that runs in your script;
To give a variable outside the parentheses, you can add something to the function that will interpret the variable to do this task. (I mentioned this detail in the last code above. 3. index)
_________________
|
|
Back to top |
|
 |
bknight2602 Grandmaster Cheater
Reputation: 0
Joined: 08 Oct 2012 Posts: 582
|
Posted: Sat Mar 29, 2025 11:38 am Post subject: |
|
|
In the code:
Code: |
Trigger(OBJECT_CAPTURE_TRIGGER, "empathabbey", "empathabbeycaptured");
function empathabbeycaptured(oldOwner,newOwner,heroName)
t_empathabbey = newOwner;
end;
|
The t_empathabbey is an object owner, just a number 1-8, that represent a player,1-8, that is used in other functions.
We don't know who will capture the object, but the function(,,heroName) should be know once the object is captured, what my thought was is to insert a function call BuildTown()before the end and have it part of that function will build the town if the playernames is part of my group. That part requires a couple of steps
playernames = GetPlayerHeroes(newOwner)--newOwner is just a number 1-8
for x = 0, length(playernames) - 1
if playernames[x] = heroName then
BuildTown(x)-- The true name of the object in the game in this case it is "empathabbey"
end;
end;
Now "empathabbey" is needed in the function
UpgradeTownBuilding(TownName, i)
In this case TownName is "empathabbey"
|
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum You cannot attach files in this forum You can download files in this forum
|
|