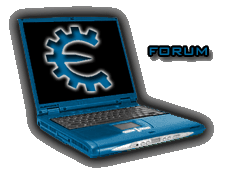 |
Cheat Engine The Official Site of Cheat Engine
|
View previous topic :: View next topic |
Author |
Message |
trifidix Newbie cheater
Reputation: 0
Joined: 11 May 2022 Posts: 16
|
Posted: Wed Dec 11, 2024 5:07 pm Post subject: running a game's own function from within lua |
|
|
hi, I want to somehow call a game's own function in memory from a lua script, including passing it a bunch of data it's supposed to work with, I'd need it to go through all its own function calls and exit when it hits a return, is that possible? I don't explicitly need the actual code already loaded into memory to run, making a copy of the function complete with all its calls, as to create an embedded assembly section in the lua script that I can work with would also be fine
|
|
Back to top |
|
 |
ParkourPenguin I post too much
Reputation: 148
Joined: 06 Jul 2014 Posts: 4593
|
Posted: Wed Dec 11, 2024 5:31 pm Post subject: |
|
|
executeCode / executeCodeEx
If it's something more advanced (e.g. esoteric ABI, or global / thread-local state needs to be modified), make a wrapper w/ autoAssemble and use executeCode on that
_________________
I don't know where I'm going, but I'll figure it out when I get there. |
|
Back to top |
|
 |
trifidix Newbie cheater
Reputation: 0
Joined: 11 May 2022 Posts: 16
|
Posted: Wed Dec 11, 2024 6:17 pm Post subject: |
|
|
I suppose executeCodeEx is indeed what I need, how do the paramaters work though? will it just push those onto the stack so that the function it's calling can grab them from there like normal? is it a normal push or do I need to worry about the stack pointer? can I directly pre-set values of registers in case I need to do that? is there any sort of issue with further function calls inside the function I'm calling with this or is it going to exit when it hits the top function's return like I want it to?
|
|
Back to top |
|
 |
ParkourPenguin I post too much
Reputation: 148
Joined: 06 Jul 2014 Posts: 4593
|
Posted: Wed Dec 11, 2024 7:43 pm Post subject: |
|
|
trifidix wrote: | I suppose executeCodeEx is indeed what I need, how do the paramaters work though? | celua.txt:
Code: | executeCodeEx(callmethod, timeout, address, {type=x,value=param1} or param1,{type=x,value=param2} or param2,...)
callmethod: 0=stdcall, 1=cdecl
timeout: Number of milliseconds to wait for a result. nil or -1, infitely. 0 is no wait (will not free the call memory, so beware of it's memory leak)
address: Address to execute
{type,value} : Table containing the value type, and the value
{
type: 0=integer (32/64bit) can also be a pointer
1=float (32-bit float)
2=double (64-bit float)
3=ascii string (will get converted to a pointer to that string)
4=wide string (will get converted to a pointer to that string)
value: anything base type that lua can interpret
}
if just param is provided CE will guess the type based on the provided type |
example:
Code: | --[[
CE Tutorial x64 step 2 write:
...
Tutorial-x86_64.exe+2B4AF - mov ecx,00000005 - parameter
Tutorial-x86_64.exe+2B4B4 - call Tutorial-x86_64.exe+FC10 - `rand` function
Tutorial-x86_64.exe+2B4B9 - add eax,01
Tutorial-x86_64.exe+2B4BC - sub [rbx+000007F8],eax - decreases health
...
]]
-- generates a random number in the interval [0,n)
function run_tutorial_rand(n)
return executeCodeEx(0, 1000, getAddress'Tutorial-x86_64.exe+FC10',{ type=0, value=n })
end
for i = 1, 10 do
print(i, '\t', run_tutorial_rand(i))
end
--[[ sample output:
1 0
2 1
3 0
4 1
5 4
6 4
7 3
8 6
9 5
10 9
]] |
trifidix wrote: | will it just push those onto the stack so that the function it's calling can grab them from there like normal? is it a normal push or do I need to worry about the stack pointer? | That depends on the calling convention. 32-bit code is a mess; 64-bit windows apps mostly use microsoft's x64 ABI.
The "callmethod" parameter for executeCodeEx determines if the callee is callee-cleanup or caller-cleanup in 32-bit code; it's ignored in 64-bit code.
TLDR: if it's 64-bit code, don't worry about it; if it's 32-bit code, you'll have to learn various calling conventions- specifically how to both recognize them in assembly and write them yourself.
trifidix wrote: | can I directly pre-set values of registers in case I need to do that? | If the callee does something against common conventions, again, you'll have to make a wrapper using autoAssemble and manage the stack & register parameters yourself.
trifidix wrote: | is there any sort of issue with further function calls inside the function I'm calling with this or is it going to exit when it hits the top function's return like I want it to? | You have to figure that out yourself. Maybe you pass a bad parameter or have the wrong global/thread state and something triggers an exception that crashes the process. Maybe there's some obfuscation and a call returns to a different place than where it was called from.
Hacking isn't an exact science. Try it and see what happens. Have backups available for when something goes terribly wrong.
_________________
I don't know where I'm going, but I'll figure it out when I get there. |
|
Back to top |
|
 |
trifidix Newbie cheater
Reputation: 0
Joined: 11 May 2022 Posts: 16
|
Posted: Wed Dec 11, 2024 8:38 pm Post subject: |
|
|
it is a 32bit program, it's a __thiscall which I'm not entirely sure how to handle, otherwise it's a fairly straight forward function which just places stuff on a map based on the input parameters which I need to call here and there and so manually setting up the stack and registers shouldn't be an issue - how do I make a wrapper with autoAssemble? I'd also like to know how does the executeCodeEx function work in the abstract sense as to wrap my head around it since it's not really explained on the wiki as far as I can see, does that actually execute whatever it finds in memory in the state that it is there, or does it create a copy while maybe adjusting offsets of the lower calls and such in the process? I've never had "real code" interact with assembly and so it's all quite confusing to me
|
|
Back to top |
|
 |
ParkourPenguin I post too much
Reputation: 148
Joined: 06 Jul 2014 Posts: 4593
|
Posted: Thu Dec 12, 2024 12:02 am Post subject: |
|
|
trifidix wrote: | it is a 32bit program, it's a __thiscall which I'm not entirely sure how to handle | Assuming you mean MSVC __thiscall, you set ecx to the instance and everything else goes through the stack as usual. The callee cleans up the stack (like stdcall). I found executeMethod which might work better in this case.
Code: | executeMethod(0, 1000, getAddress'game.exe+1234', 0x22D4178C0, {type=x, value=param1}, ...) |
trifidix wrote: | how do I make a wrapper with autoAssemble? I'd also like to know how does the executeCodeEx function work in the abstract sense |
- Allocate some memory in the target process
- Write to that memory some instructions that call a function and return
- Create a thread that executes those instructions
- Wait for the thread to finish
- Deallocate the memory
An auto assemble script can handle just about all of that. createthread info:
https://forum.cheatengine.org/viewtopic.php?t=619046
Lua would be used to enable and disable the script, handling the disable info in the process. (if disabling the script is even needed)
_________________
I don't know where I'm going, but I'll figure it out when I get there. |
|
Back to top |
|
 |
trifidix Newbie cheater
Reputation: 0
Joined: 11 May 2022 Posts: 16
|
Posted: Thu Dec 12, 2024 11:09 pm Post subject: |
|
|
oh so executeMethod alone does in fact work without needing to get any assembly involved, is this explicitly meant for msvc __thiscall, looking at how it assumes ecx if no register number is provided? anyway many thanks for explaining this to me, it'll probably save me a thousand hours of work
|
|
Back to top |
|
 |
ParkourPenguin I post too much
Reputation: 148
Joined: 06 Jul 2014 Posts: 4593
|
Posted: Fri Dec 13, 2024 12:19 am Post subject: |
|
|
trifidix wrote: | looking at how it assumes ecx if no register number is provided | Yes. See celua.txt in the main CE directory for some basic documentation.
_________________
I don't know where I'm going, but I'll figure it out when I get there. |
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum You cannot attach files in this forum You can download files in this forum
|
|