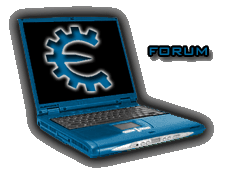 |
Cheat Engine The Official Site of Cheat Engine
|
View previous topic :: View next topic |
Author |
Message |
Game Hacking Dojo Expert Cheater
Reputation: 1
Joined: 17 Sep 2023 Posts: 110
|
Posted: Tue May 07, 2024 10:00 am Post subject: Fixing Seach Function |
|
|
I've made a messy code that partially works but I want to improve it.
My vision of the script:
- it has to search a directory and its subdirectories for the file and file extension passed
- If it finds 1 stop the search. if more than 1 occurrence were found in the directory and subdirectories of the first occurrence then let me do something and stop the search.
- I want the search function to be universal if possible.
What happens now:
- it finds the file and keeps running.
I want it to find the file stop, search the last directory and subdirectories and return the number of occurrences.
And thank you for helping. I am learning thanks to you.
Code: | local lfs = require("lfs")
local directory = "D:\\mydirectory\\mysubdirectory\\Game Hacks"
local fileExtension = ".ct"
local filePath = ""
local file = ""
local function searchFile(directory, fileName, fileExtension, searchType)
local fileFoundCount = 0 -- Variable to count occurrences of the file
for file in lfs.dir(directory) do -- Iterate over the contents of the directory
if file ~= "." and file ~= ".." then
local filePath = directory.. "\\" .. file -- Construct the full file path
print(filePath)
local attr = lfs.attributes(filePath)
if attr.mode == "directory" then -- Check if the current item is a directory
searchFile(filePath, fileName, fileExtension) -- If it's a directory, recursively search it
elseif string.find(string.lower(file), string.lower(fileName), 1, true) and string.find(string.lower(file), string.lower(fileExtension), 1, true) then
fileFoundCount = fileFoundCount + 1 -- Increment the count if file is found in current directory
filePath = filePath:gsub(file, "", nil, true)
print("Found file name: " .. file)
print("Found file path: " .. filePath)
print("Found file count: " .. fileFoundCount)
print("Searching culprit directory:")
searchFile(filePath, fileName, fileExtension)
return
end
end
end
end
function searchCT(directory)
processName = process:gsub("%.exe", "")..fileExtension
searchFile(directory, processName, fileExtension)
print("*** Done ***")
end |
|
|
Back to top |
|
 |
ParkourPenguin I post too much
Reputation: 143
Joined: 06 Jul 2014 Posts: 4371
|
Posted: Tue May 07, 2024 1:29 pm Post subject: |
|
|
CE's Lua API has some relevant functions. celua.txt:
Quote: | getFileList(Path:string, searchMask:string OPTIONAL, SearchSubDirs: boolean OPTIONAL, DirAttrib: integer OPTIONAL): Returns an indexed table with filenames
getDirectoryList(Path:string, SearchSubDirs: boolean OPTIONAL): Returns an indexed table with directory names
extractFileName(filepath): returns the filename of the path
extractFileExt(filepath): returns the file extension of the path
extractFileNameWithoutExt(filepath): Returns the filename of the path, without the extension
extractFilePath(filepath): removes the filename from the path
getTempFolder() : Returns the path to the temp folder
fileExists(pathtofile): Returns true if a file exists at that path
deleteFile(pathtofile): Returns true if a file existed at that path, and now not anymore
|
Example:
Code: | -- print all .ct files in a certain directory recursively
local path = [[D:\mydirectory\mysubdirectory\Game Hacks]]
for _,p in ipairs(getFileList(path, '*.ct', true)) do
print(p)
end |
_________________
I don't know where I'm going, but I'll figure it out when I get there. |
|
Back to top |
|
 |
AylinCE Grandmaster Cheater Supreme
Reputation: 33
Joined: 16 Feb 2017 Posts: 1329
|
Posted: Tue May 07, 2024 2:56 pm Post subject: |
|
|
I fixed the existing code, but @ParkourPenguin's idea is more stable, concise and understandable.
Here's whichever you want;
Code: | local directory = [[D:\mydirectory\mysubdirectory\Game Hacks\]]
local fileExtension = ".ct"
local filePath = ""
local file = ""
local function searchFile(directory, fileName, fileExtension, searchType)
local fileFoundCount = 0
local filePath1 = ""
fileExtension = (fileExtension):lower()
for file in lfs.dir(directory) do
local Path1 = directory.. "\\" .. file
--print("Found file: " .. file)
local attr = lfs.attributes(Path1)
if attr.mode == "directory" then
filePath1 = directory.. "\\" .. file
--print("Found file path: " .. filePath1)
else
filePath = filePath.. "\\" .. file
fileFoundCount = fileFoundCount + 1
ext = filePath:match("^.+(%..+)$"):lower()
foundName = extractFileName(filePath)
foundName = foundName:gsub("...$","")
--print("Found file name: " .. foundName.." - "..ext)
if ext==fileExtension then
if foundName==fileName then
print("Found file path: " .. filePath1)
print("Found file name: " .. foundName)
print("Found file extension: " .. ext)
print("Found file count: " .. fileFoundCount)
return
end
else
filePath1 = directory
--It does not include the last read folder in the current path.
--This prevents; Path (folder1, folder2, folder3, name.ct)
--Result: Path-folder3-name.ct
--Correct path: Path-name.ct
end
end
end
end
function searchCT(directory)
processName = process:gsub("%.exe", "") --..fileExtension
searchFile(directory, processName, fileExtension)
print("*** Done ***")
end
searchCT(directory) |
-- or
Code: | local directory = [[D:\mydirectory\mysubdirectory\Game Hacks\]]
function searchFile(directory, fileName, fileExtension)
local fileFoundCount=0
for _,p in ipairs(getFileList(directory, '*'..fileExtension, true)) do
fileFoundCount = fileFoundCount + 1
foundName = extractFileName(p)
--print(foundName)
ext = foundName:match("^.+(%..+)$"):lower()
foundName = foundName:gsub("...$","")
filePath1 = extractFilePath(p)
--print("Found file name: " .. foundName.." - "..ext)
if ext==fileExtension then
if foundName==fileName then
print("Found file path: " .. filePath1)
print("Found file name: " .. foundName)
print("Found file extension: " .. ext)
print("Found file count: " .. fileFoundCount)
--return
end
end
end
end
function searchCT(directory)
processName = process:gsub("%.exe", "") --..fileExtension
searchFile(directory, processName, ".ct")
print("*** Done ***")
end
searchCT(directory) |
_________________
|
|
Back to top |
|
 |
Game Hacking Dojo Expert Cheater
Reputation: 1
Joined: 17 Sep 2023 Posts: 110
|
Posted: Wed May 08, 2024 4:01 am Post subject: |
|
|
Thank you guys for helping I really appreciate it.
|
|
Back to top |
|
 |
|
|
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum You cannot attach files in this forum You can download files in this forum
|
|